Terraform Security Best Practices: A beginner’s Guide to a IaC Setup
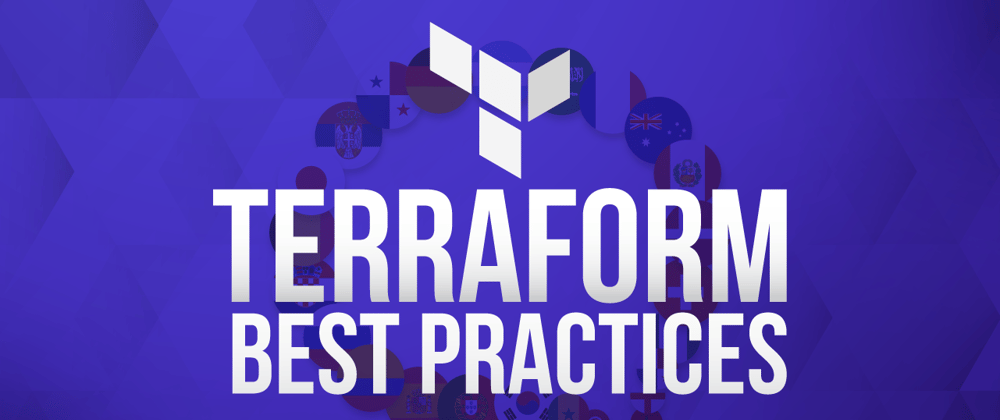
Terraform Security Best Practices:
When managing cloud infrastructure using Terraform, ensuring security is not optional; it’s a critical component of a reliable Infrastructure as Code (IaC) strategy. Mistakes, such as exposing sensitive information or misconfiguring permissions, can lead to severe vulnerabilities and costly breaches.
This guide outlines essential security practices for Terraform, including effective secrets management, state file protection, and secure module design. By implementing these strategies, you can establish a robust and secure foundation for your Terraform projects.
Here’s a step-by-step guide to keeping your Terraform setup secure.
1. Secrets Management: Securing Sensitive Data
One common mistake is hardcoding secrets (like API keys) directly into .tf
files.
Scenario:
Imagine you are configuring an S3 bucket in Terraform. Rather than hardcoding your AWS credentials directly in your Terraform files, you store them in HashiCorp Vault and retrieve them dynamically during the Terraform deployment process.
This approach minimizes the risk of credential exposure while keeping your infrastructure secure.
Store Secrets in Vault
Store your AWS credentials securely in Vault:
bash
Copy code
vault kv put secret/aws creds='{"access_key": "AKIA...","secret_key": "wJal..."}'
Step 2: Retrieve Secrets Dynamically in Terraform
main.tf
hcl
Copy code
provider "vault" {
address = "https://vault.yourdomain.com"
}
data "vault_kv_secret_v2" "aws_creds" {
mount = "secret"
name = "aws"
}
provider "aws" {
access_key = jsondecode(data.vault_kv_secret_v2.aws_creds.data["creds"]).access_key
secret_key = jsondecode(data.vault_kv_secret_v2.aws_creds.data["creds"]).secret_key
region = var.region
}
resource "aws_s3_bucket" "example" {
bucket = "secure-bucket-example"
acl = "private"
}
variables.tf
hcl
Copy code
variable "region" {
description = "AWS region"
type = string
default = "us-east-1"
}
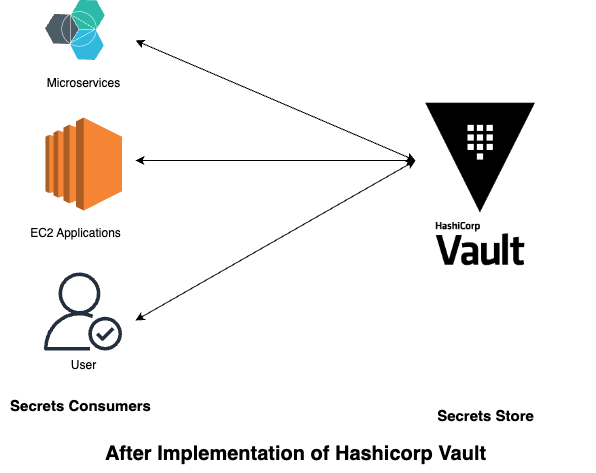
Security Measures
- Secrets in Vault: Credentials are stored securely and never exposed in
.tf
files or version control. .gitignore
: Ensureterraform.tfstate
and any.tfvars
are excluded from version control to protect sensitive data.- Dynamic Retrieval: Secrets are fetched dynamically during execution, reducing the risk of leaks and accidental exposure.
This method of using HashiCorp Vault with Terraform ensures that your sensitive data is securely managed while maintaining flexibility in your infrastructure deployments.
2. State File Security: Protecting Terraform State
Terraform’s state file contains critical information about your infrastructure. If compromised, it could lead to unauthorized changes or data leaks.
Hypothetical Scenario:
In this scenario, you configure an S3 bucket for storing Terraform state files, ensuring that server-side encryption is enabled. Additionally, you apply an IAM policy to restrict access to the state file to authorized users only.
Step 1: Configure S3 for Remote State Storage
backend.tf
hcl
Copy code
terraform {
backend "s3" {
bucket = "my-terraform-state"
key = "terraform.tfstate"
region = "us-east-1"
encrypt = true
acl = "private"
dynamodb_table = "terraform-locks"
}
}
Step 2: Configure IAM Policy for Role-Based Access Control
iam-policy.json
json
Copy code
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"s3:GetObject",
"s3:PutObject"
],
"Resource": "arn:aws:s3:::my-terraform-state/*"
},
{
"Effect": "Deny",
"Action": [
"s3:DeleteObject"
],
"Resource": "arn:aws:s3:::my-terraform-state/*"
}
]
}
aws_iam_policy
hcl
Copy code
resource "aws_iam_policy" "state_access_policy" {
name = "TerraformStateAccessPolicy"
policy = file("iam-policy.json")
}
resource "aws_iam_role_policy_attachment" "role_policy_attachment" {
role = "my-team-role"
policy_arn = aws_iam_policy.state_access_policy.arn
}
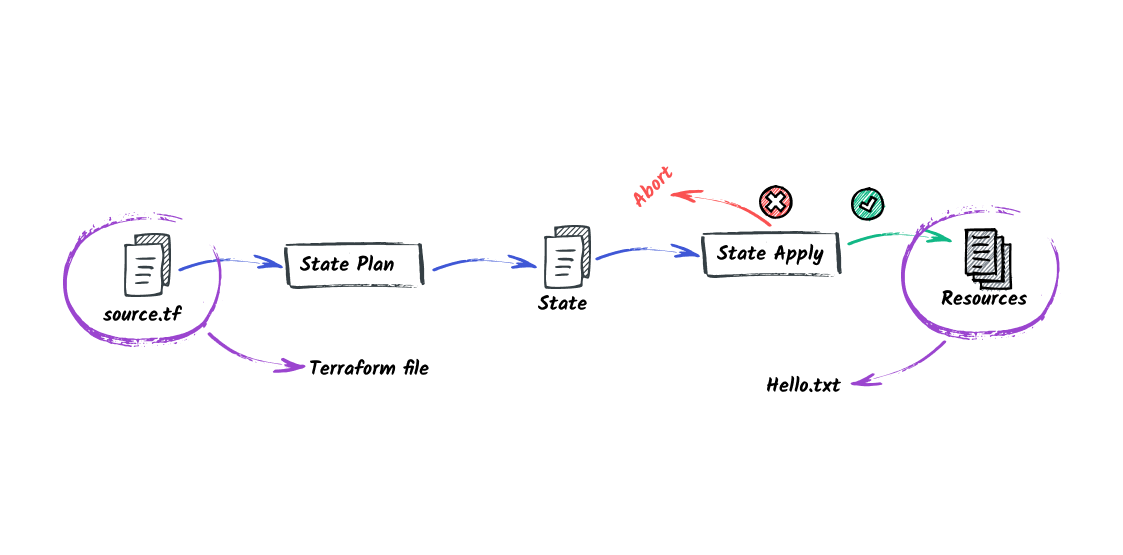
Key Security Measures
- Remote State Storage with Encryption: By using a secure, remote backend (S3) with encryption enabled, you ensure that sensitive data within the state file is protected both at rest and in transit.
- Access Control via RBAC: Fine-grained IAM policies restrict access to state files, ensuring that only authorized users can modify or access the state file.
- State Locking and Versioning: Enabling state locking (via DynamoDB) prevents multiple users from modifying the state concurrently while versioning ensures you can recover from mistakes or accidental data loss.
By following these practices and securing your state file, you can prevent unauthorized access, accidental data loss, and ensure that your infrastructure remains safe and reliable.
3. Module Design: Creating Secure Modules
Modules are the building blocks of Terraform configurations. Poorly designed modules can lead to vulnerabilities.
Scenario:
You create a module for deploying EC2 instances. Instead of granting broad IAM permissions, your module only allows actions like starting and stopping instances, following the principle of least privilege.
Module Design for EC2 Instances with Least Privilege
In this example, we’re creating a Terraform module for deploying EC2 instances with security best practices. By following the principle of least privilege, the module grants only necessary permissions (e.g., start and stop instances), reducing the risk of unauthorized actions.
This module includes key elements for secure usage: a minimal IAM policy, reusable inputs and outputs, and thorough documentation.
main.tf
resource "aws_instance" "ec2" {
ami = var.ami_id
instance_type = var.instance_type
iam_instance_profile = aws_iam_instance_profile.ec2_profile.id
tags = { Name = var.instance_name }
}
resource "aws_iam_role" "ec2_role" {
name = "${var.instance_name}-role"
assume_role_policy = jsonencode({
Version = "2012-10-17",
Statement = [{
Action = "sts:AssumeRole",
Effect = "Allow",
Principal = { Service = "ec2.amazonaws.com" }
}]
})
}
resource "aws_iam_policy" "ec2_policy" {
name = "${var.instance_name}-policy"
policy = jsonencode({
Version = "2012-10-17",
Statement = [{
Action = ["ec2:StartInstances", "ec2:StopInstances"],
Effect = "Allow",
Resource = "*"
}]
})
}
resource "aws_iam_role_policy_attachment" "attach_policy" {
role = aws_iam_role.ec2_role.name
policy_arn = aws_iam_policy.ec2_policy.arn
}
resource "aws_iam_instance_profile" "ec2_profile" {
name = "${var.instance_name}-profile"
role = aws_iam_role.ec2_role.name
}
variables.tf
hcl
Copy code
variable "ami_id" { type = string }
variable "instance_type" { type = string; default = "t2.micro" }
variable "instance_name" { type = string }
outputs.tf
hcl
Copy code
output "instance_id" { value = aws_instance.ec2.id }
output "instance_iam_role" { value = aws_iam_role.ec2_role.name }
Key Highlights
- Principle of Least Privilege: Only grants
ec2:StartInstances
andec2:StopInstances
actions, limiting potential exposure. - Reusable and Documented: Modular design with well-defined variables and outputs for ease of use and clarity.
This example illustrates secure module design by focusing on minimal permissions and effective documentation, providing a solid foundation for deploying infrastructure with Terraform.
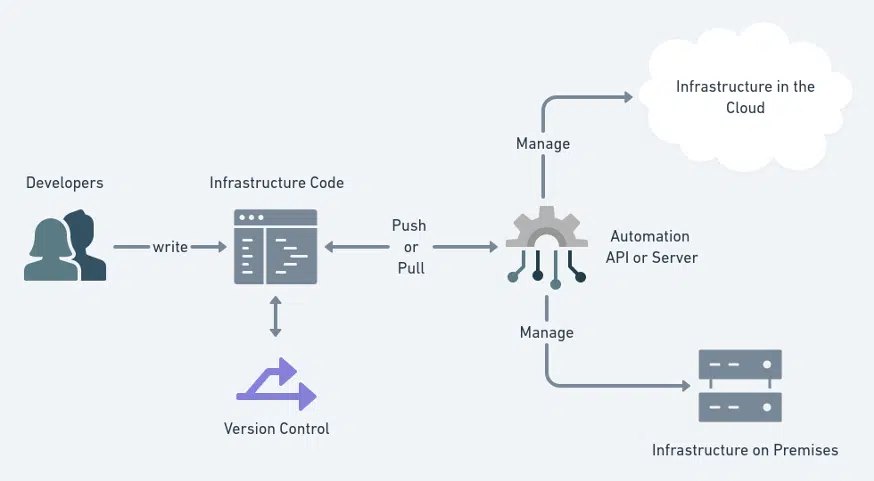
4. Version Control: Protecting the Codebase
Version control systems like Git are indispensable, but they can be a double-edged sword if misused.
Scenario:
You set up a pre-commit hook that checks for hardcoded secrets and other security missteps before pushing code to the repository.
Step 1: Add Sensitive Files to .gitignore
.gitignore
gitignore
Copy code
# Exclude Terraform state files
*.tfstate
*.tfstate.backup
# Exclude variable definition files
*.tfvars
# Exclude any other sensitive files
*.pem
*.key
Step 2: Set Up TFSec Pre-Commit Hook
- Install pre-commit and TFSec.
bash
Copy code
pip install pre-commit
brew install tfsec
- Configure the pre-commit hook for TFSec:
.pre-commit-config.yaml
yaml
Copy code
- repo: https://github.com/aquasecurity/tfsec
rev: v1.30.0 # Use the latest stable version
hooks:
- id: tfsec
name: tfsec - Terraform security scanner
language: system
- Install the pre-commit hook:
bash
Copy code
pre-commit install
Step 3: Run Pre-Commit Hook
Now, when you attempt to commit any changes, TFSec will automatically scan your Terraform files for potential security issues, such as hardcoded secrets or misconfigurations.
bash
Copy code
git commit -m "Fix security issue"
If any issues are found, the commit will be blocked, allowing you to address the problems before pushing the code to the repository.
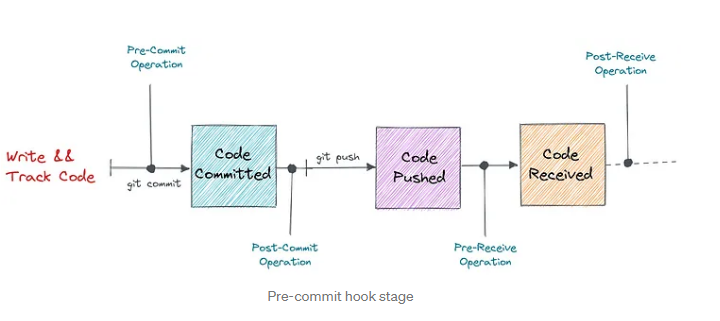
Key Security Measures:
.gitignore
for Sensitive Files: Ensure that Terraform state files and other sensitive data (e.g.,.tfvars
, credentials) are never accidentally committed to version control.- Pre-Commit Hooks for Automated Scanning: TFSec provides an automated way to identify security issues in Terraform code before changes are pushed to the repository, catching potential misconfigurations early.
- Pull Request Workflow: Implementing a PR review process ensures that changes are scrutinized by other team members, which can help identify potential security risks before code is merged.
By implementing these best practices, you can ensure that your Terraform codebase remains secure, minimizing the risk of accidental leaks or security misconfigurations.
5. Plan and Apply Safely: Controlling Deployments
Terraform’s plan
and apply
commands are powerful but can also be dangerous if used recklessly.
Best Practices:
- Review Plans Carefully: Always review the output of
terraform plan
to ensure no unintended changes are made. - Lock Resources: Use
terraform state lock
to prevent concurrent updates to your infrastructure. - Automate with CI/CD: Integrate Terraform into a CI/CD pipeline to enforce consistent workflows and catch issues early.
Scenario:
In this scenario, you automate the process of reviewing the output of terraform plan
through your CI/CD pipeline to detect potential anomalies before applying changes to your infrastructure.
Step 1: Configure Terraform Plan in CI/CD Pipeline
You can use GitHub Actions, GitLab CI, or Jenkins to automate Terraform execution. Here's an example using GitHub Actions:
.github/workflows/terraform.yml
yaml
Copy code
name: Terraform Plan and Apply
on:
pull_request:
branches:
- main
jobs:
terraform:
runs-on: ubuntu-latest
steps:
- name: Checkout repository
uses: actions/checkout@v2
- name: Set up Terraform
uses: hashicorp/setup-terraform@v1
- name: Terraform Init
run: terraform init
- name: Terraform Plan
id: plan
run: terraform plan -out=tfplan
- name: Review Plan Output
run: |
terraform show -no-color tfplan | tee plan.txt
if grep -q "Destroy" plan.txt; then
echo "Plan includes resource destruction, aborting the deployment."
exit 1
fi
- name: Terraform Apply (if safe)
run: terraform apply -auto-approve tfplan
if: success()
Step 2: Lock Resources with State Locking
To prevent concurrent updates, enable state locking using DynamoDB (for AWS S3 backend) or equivalent in other cloud platforms.
backend.tf
hcl
Copy code
terraform {
backend "s3" {
bucket = "my-terraform-state"
key = "terraform.tfstate"
region = "us-east-1"
encrypt = true
dynamodb_table = "terraform-locks" # Enables state locking
}
}
Step 3: Automate terraform plan
Review and Prevent Anomalies
In the CI/CD pipeline, the plan output is reviewed to ensure no unintended changes, such as resource deletions, are included.
- Plan Review: The plan output is parsed, and any references to resource destruction (e.g., "Destroy") will cause the job to fail, preventing accidental deletions.
- State Locking: The backend configuration ensures that only one process can modify the state at a time, preventing race conditions.
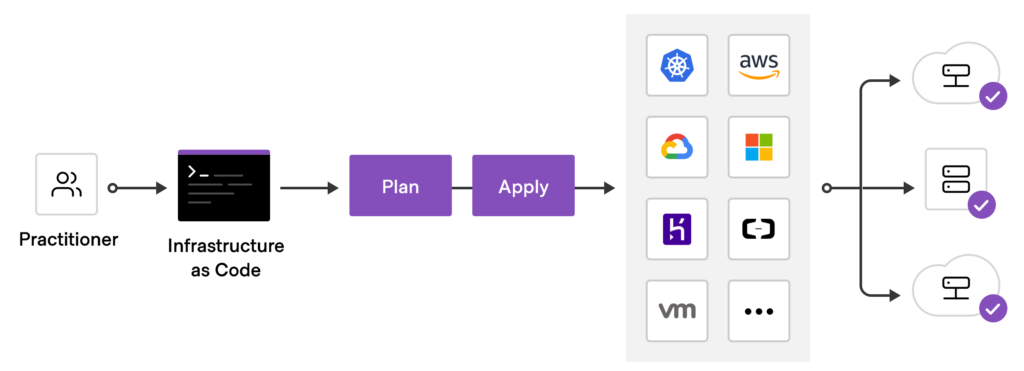
Key Security Measures:
- Review Plans Carefully: Automating the
terraform plan
review ensures that no unintended infrastructure changes (e.g., deletions) are applied, reducing human error. - State Locking: Locking the state file prevents concurrent modifications, avoiding potential issues with simultaneous
terraform apply
commands. - CI/CD Integration: Using a CI/CD pipeline ensures that Terraform commands are executed in a controlled environment, enforcing consistent workflows and reducing the risk of manual mistakes.
By automating Terraform workflows and incorporating safeguards, you can ensure that your infrastructure is deployed safely and predictably.
6. Continuous Monitoring: Ensuring Proactive Security
Security doesn’t end after deployment. Continuously monitor and audit your Terraform setup.
Best Practices:
- Automated Scanning: Use tools like Checkov or TFSec to identify misconfigurations.
- Auditing: Regularly review logs and state file changes to detect suspicious activity.
- Policy as Code: Implement guardrails using tools like Sentinel or Open Policy Agent (OPA) to enforce security policies.
Scenario:
In this scenario, you set up TFSec to automatically scan your Terraform code for security issues and notify your team if any high-risk issues are detected.
Step 1: Configure TFSec for Automated Scanning
You can set up TFSec to automatically run as part of your CI/CD pipeline to scan for vulnerabilities.
.github/workflows/terraform-security.yml
yaml
Copy code
name: Terraform Security Scan
on:
push:
branches:
- main
pull_request:
branches:
- main
jobs:
terraform-security-scan:
runs-on: ubuntu-latest
steps:
- name: Checkout repository
uses: actions/checkout@v2
- name: Set up Terraform
uses: hashicorp/setup-terraform@v1
- name: Install TFSec
run: curl -s https://raw.githubusercontent.com/aquasecurity/tfsec/master/scripts/install.sh | bash
- name: Run TFSec Security Scan
run: |
tfsec . --format=github-actions
if [ $? -ne 0 ]; then
echo "TFSec found security issues, aborting!"
exit 1
fi
- name: Notify Team
if: failure()
run: |
curl -X POST -H 'Content-Type: application/json' \
-d '{"text":"TFSec detected critical issues in the Terraform code."}' \
YOUR_SLACK_WEBHOOK_URL
Step 2: Enable Logging for Auditing
Enable logging for Terraform runs, such as the apply
and plan
logs, to track changes to your infrastructure over time.
Example: Store Logs in AWS CloudWatch
hcl
Copy code
resource "aws_cloudwatch_log_group" "terraform_log_group" {
name = "/aws/terraform/logs"
}
resource "aws_cloudwatch_log_stream" "terraform_log_stream" {
name = "terraform_logs"
log_group_name = aws_cloudwatch_log_group.terraform_log_group.name
}
resource "aws_cloudwatch_log_subscription_filter" "terraform_log_filter" {
name = "terraform_log_filter"
log_group_name = aws_cloudwatch_log_group.terraform_log_group.name
filter_pattern = ""
destination_arn = "arn:aws:sns:us-east-1:123456789012:terraform-logs"
}
This will help you capture any suspicious or unauthorized changes to the infrastructure and review them regularly.
Step 3: Implement Policy as Code with Sentinel or OPA
For proactive security, you can use Sentinel or Open Policy Agent (OPA) to enforce security policies before changes are applied.
Example: Implement Sentinel Policy for Terraform
hcl
Copy code
# Sentinel Policy to enforce S3 bucket encryption
import "tfplan/v2" as tfplan
main = rule {
all tfplan.resources.aws_s3_bucket as _, bucket {
bucket.applies = bucket.configuration.encryption != null
}
}
This policy ensures that all S3 buckets must have encryption enabled before changes are applied.
Key Security Measures:
- Automated Scanning: Tools like TFSec ensure that vulnerabilities in Terraform code are automatically detected during the CI/CD process, helping identify risks before deployment.
- Auditing Logs: Storing logs in services like AWS CloudWatch allows you to track infrastructure changes and detect suspicious activity over time.
- Policy as Code: Enforcing security policies using tools like Sentinel or OPA helps prevent deploying insecure resources by enforcing predefined security standards.
By continuously monitoring your Terraform setup with these tools, you can catch security misconfigurations early and prevent potential vulnerabilities from affecting your infrastructure.
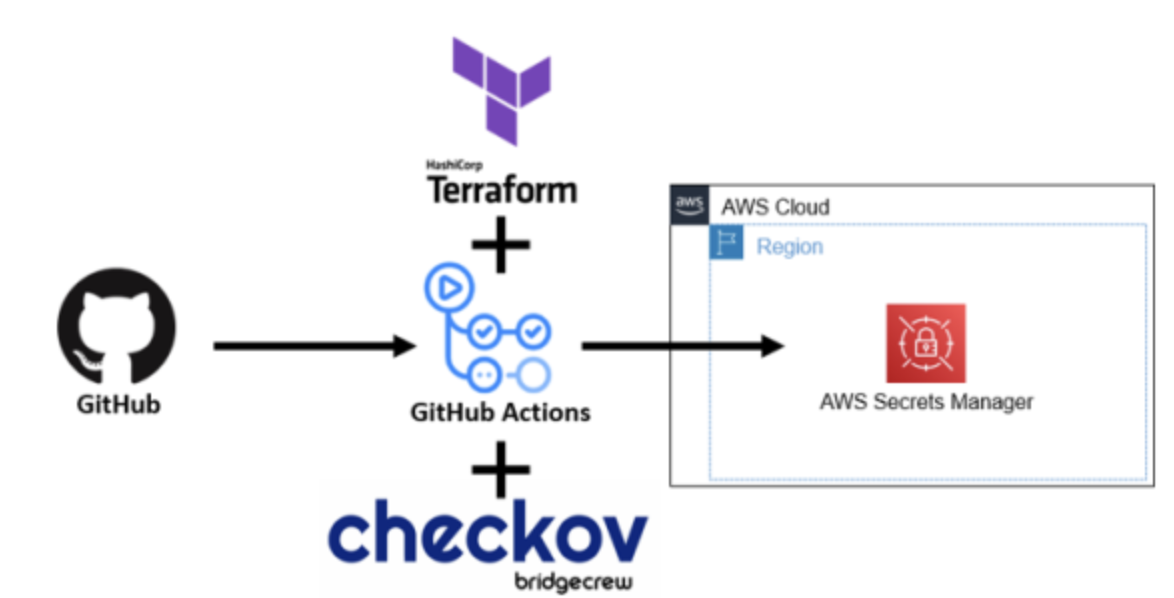
Simplify and Secure Terraform with Scoutflo
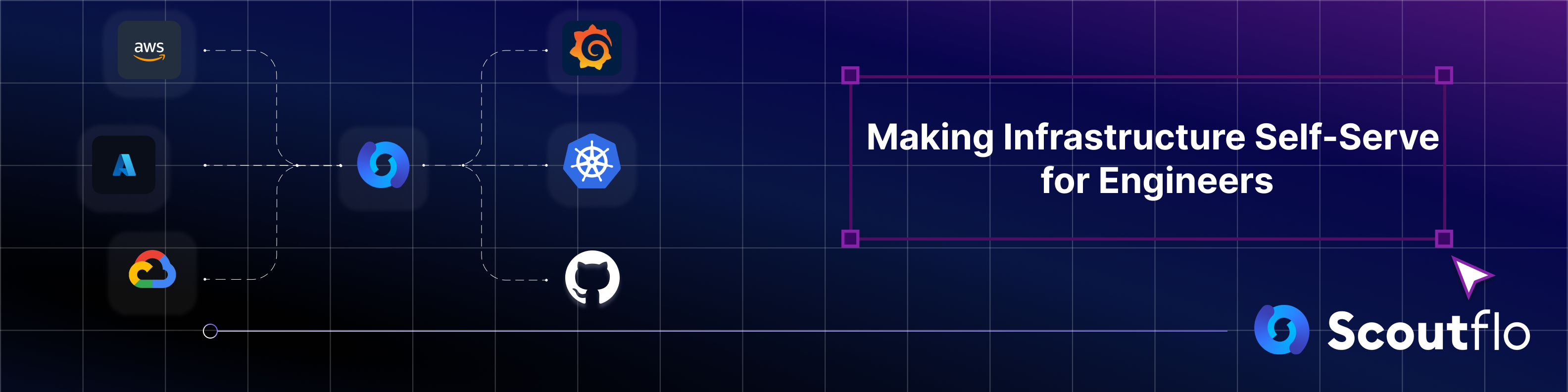
Managing Terraform infrastructure securely requires attention to detail and adherence to best practices. However, this process can become complex and time-consuming, especially for teams working in dynamic cloud environments.
With Scoutflo, an advanced Infrastructure as Code (IaC) management platform, you can:
- Automate Workflows: Streamline Terraform workflows with built-in CI/CD integrations and automated approval processes.
- Enhance Security: Enforce policies as code, manage secrets securely, and track every Terraform plan and apply with comprehensive audit logs.
- Collaborate Efficiently: Enable role-based access control (RBAC), self-service deployments, and environment governance across teams.
Scoutflo allows you to manage and secure your Terraform infrastructure with ease, so your team can focus on innovation without compromising security.
Sign up here to get started with Terraform!
And don’t forget to follow Scoutflo on Twitter if you haven’t already! ✨
We’re also active on LinkedIn 💙